Q Learning with Gym
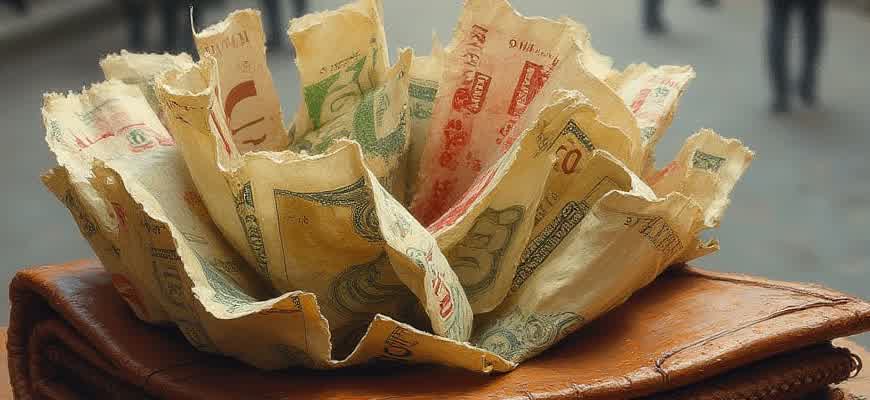
Q-learning is a widely used reinforcement learning algorithm that enables an agent to learn optimal actions through interaction with its environment. By using Q-learning, an agent can explore its environment and update its policy based on received rewards. OpenAI Gym provides a platform for developing and testing reinforcement learning algorithms by simulating different environments.
The process of applying Q-learning within Gym involves the following steps:
- Initialize a Q-table with zeros.
- At each step, the agent chooses an action based on an epsilon-greedy policy.
- Perform the chosen action, observe the resulting state and reward.
- Update the Q-value for the state-action pair based on the observed reward and the estimated future rewards.
- Repeat the process until convergence or the maximum number of episodes is reached.
Important Note: The Q-table stores the expected future reward for each action in a given state. This table is updated iteratively as the agent explores the environment.
To better understand how Q-learning is implemented with Gym, let's consider an example:
State | Action | Reward | Next State |
---|---|---|---|
S0 | A1 | +1 | S1 |
S1 | A2 | -1 | S2 |
S2 | A3 | +2 | S3 |
Setting Up Your Environment for Q Learning in OpenAI Gym
Before starting with Q Learning, it is essential to configure the proper environment in OpenAI Gym. This process includes installing necessary libraries, understanding the environment setup, and choosing the right environment for your task. Proper configuration ensures that you can successfully apply Q Learning algorithms to solve reinforcement learning problems.
To begin, you will need to install key libraries, set up the Gym environment, and initialize a suitable environment for your experiment. Below is a guide to ensure you are ready to begin working with Q Learning in OpenAI Gym.
Step-by-Step Setup
- Install Required Libraries
- Install Python packages like
gym
andnumpy
:
pip install gym numpy
- Install Python packages like
- Optional: Install extra dependencies for specific environments (e.g.,
atari
for games): - Create the Gym Environment
- Choose a suitable environment for your learning problem. Gym offers various environments like
CartPole-v1
,MountainCar-v0
, and many others. - Example code to create an environment:
import gym env = gym.make("CartPole-v1")
- Choose a suitable environment for your learning problem. Gym offers various environments like
- Set Up Your Q Learning Algorithm
- Define the action space, state space, and reward structure for the chosen environment.
- Initialize the Q-table for state-action values.
pip install gym[atari]
Important: Ensure that you select an environment that matches your learning goals. For instance, CartPole-v1
is a popular choice for beginners, as it has discrete actions and simple state space.
Configuring Environment Variables
When setting up Gym, you may encounter certain environment variables that require configuration. Here’s a basic table with commonly used environment variables:
Variable | Description |
---|---|
GYM_USE_GPU | Determines whether GPU acceleration is used. Set to True if you want faster training on supported environments. |
GYM_DISABLE_ENV | Disables specific environments from loading, useful for debugging. |
OPENAI_LOG_FORMAT | Defines the logging format for Gym during training. Helps to track the learning progress and results. |
Choosing the Right Q-Learning Algorithm for Your Project
When implementing Q-learning in a project, selecting the most suitable algorithm is crucial for achieving optimal results. The choice of algorithm largely depends on the environment, the complexity of the problem, and the available computational resources. Different versions of Q-learning, such as tabular Q-learning, Deep Q-Networks (DQN), and Double Q-learning, offer unique advantages and limitations that can impact your model's efficiency and performance.
Understanding the strengths and weaknesses of various Q-learning approaches can help streamline the decision-making process. In this section, we will explore the core considerations when choosing a Q-learning variant, and provide a comparison of different algorithms to guide you in selecting the best one for your project needs.
Key Factors to Consider
- Problem Complexity: Simple environments with discrete states and actions may work well with basic Q-learning, while more complex, high-dimensional problems may require Deep Q-Networks (DQN).
- Memory and Computational Efficiency: Tabular Q-learning is computationally efficient but not scalable for large state-action spaces. In contrast, DQN handles larger spaces but at the cost of higher computational power.
- Exploration vs. Exploitation: Algorithms like Double Q-learning help mitigate overestimation of Q-values, leading to better performance in environments with high uncertainty.
Algorithm Comparison Table
Algorithm | Strengths | Weaknesses |
---|---|---|
Tabular Q-Learning | Simple to implement, fast for small environments | Not scalable for large state-action spaces |
DQN | Handles high-dimensional spaces, effective for complex problems | Requires more computational power, may need tuning for stability |
Double Q-Learning | Reduces overestimation bias, improved stability | More computationally intensive than standard Q-learning |
Important: For environments with a large number of states, a deep Q-network (DQN) may be the better option due to its ability to generalize using neural networks, which tabular methods cannot handle effectively.
Understanding the Exploration vs. Exploitation Dilemma in Q Learning
In Q Learning, an agent faces a fundamental decision at each step: whether to explore new actions or to exploit the knowledge it has already acquired. This decision, known as the *exploration-exploitation trade-off*, has a significant impact on the agent’s ability to optimize its learning process. Balancing between exploring new strategies and exploiting existing ones is crucial for an agent to maximize long-term rewards while minimizing unnecessary computational effort.
Exploration refers to trying out new actions that might lead to unknown states, whereas exploitation involves selecting the action that is currently believed to provide the highest reward based on the agent’s experience. This dilemma is typically managed through an exploration policy, such as epsilon-greedy, which determines the likelihood of choosing a random action (exploration) versus the best-known action (exploitation). However, the balance must be carefully adjusted to avoid premature convergence to suboptimal solutions or excessive randomness.
Strategies for Balancing Exploration and Exploitation
To solve this dilemma, several approaches are utilized in Q Learning. Here are some common strategies:
- Epsilon-greedy: Selects the best-known action with probability (1 - epsilon) and a random action with probability epsilon.
- Softmax: Probabilistically selects actions based on the estimated Q-values, favoring higher-value actions without fully exploiting them.
- Upper Confidence Bound (UCB): Balances exploration and exploitation by selecting actions that have the highest upper confidence bound on their Q-values.
Impact of Exploration vs. Exploitation on Learning
Properly managing the trade-off directly influences how quickly and effectively an agent learns the optimal policy. Below is a summary of key factors involved in the decision-making process:
Factor | Effect of Exploration | Effect of Exploitation |
---|---|---|
Long-Term Optimality | Helps discover the best possible actions over time | May lead to suboptimal policies if exploration is insufficient |
Convergence Speed | Slower, as more time is spent testing different actions | Faster, as the agent focuses on known successful actions |
Exploration Efficiency | May result in redundant exploration if not controlled | More efficient once a reliable policy is found |
In Q Learning, the agent’s ability to find the optimal balance between exploration and exploitation significantly impacts the quality of the learned policy.
Fine-Tuning Hyperparameters for Enhanced Performance in Gym
When implementing Q-learning algorithms with Gym environments, achieving optimal performance often depends on the careful adjustment of hyperparameters. These parameters control the learning process, impacting how quickly and effectively the agent can solve tasks. While many default values are available, fine-tuning these settings can result in faster convergence and higher rewards in more complex environments. Key hyperparameters like learning rate, discount factor, exploration strategy, and the number of episodes should be adjusted based on the task and environment characteristics.
Effective hyperparameter tuning requires a structured approach to test and modify settings iteratively. Without careful adjustments, the agent may either fail to learn efficiently or overfit to the environment. This process often involves balancing exploration and exploitation, adjusting the model's learning speed, and ensuring the agent receives appropriate feedback. Below are essential hyperparameters that should be considered when aiming for improved Q-learning performance in Gym environments.
Key Hyperparameters to Tune
- Learning Rate (α): Determines the extent to which new information overrides old. A smaller value results in slow learning, while a larger value may cause instability.
- Discount Factor (γ): Controls the importance of future rewards. A high discount factor encourages long-term planning, while a low factor focuses on immediate rewards.
- Exploration vs. Exploitation (ε): Sets the probability of the agent choosing a random action (exploration) versus the action with the highest Q-value (exploitation). A typical approach is to decrease exploration over time.
- Episodes and Steps per Episode: The number of episodes and steps per episode determine how long the agent trains and how many decisions it makes. A higher number provides more training opportunities but may increase computation time.
Practical Tuning Tips
- Gradual ε Decay: Begin with a high exploration rate and decrease it over time to allow the agent to shift from exploration to exploitation gradually.
- Adjust Learning Rate Dynamically: If the agent's performance stagnates, consider reducing the learning rate to avoid overshooting optimal values.
- Monitor Rewards: Track the total rewards per episode. A decrease in rewards may indicate a need to revisit the discount factor or learning rate.
- Test with Multiple Environments: Fine-tune parameters on different Gym environments to ensure robustness in diverse scenarios.
Tip: Hyperparameters like α and γ should be tested across a range of values to understand their impact on different environments, as their optimal values often vary between tasks.
Sample Hyperparameter Table
Hyperparameter | Typical Range | Recommended Value |
---|---|---|
Learning Rate (α) | 0.001 - 0.1 | 0.01 |
Discount Factor (γ) | 0.8 - 0.99 | 0.95 |
Exploration Rate (ε) | 0.1 - 1.0 | 0.9 (decay to 0.1 over time) |
Episodes | 1000 - 10000 | 5000 |
Improving Q-Learning with Reward Shaping
Reward shaping is a technique used to modify the reward signal provided to an agent in reinforcement learning to enhance the speed and efficiency of learning. By adjusting the reward function, agents can be steered towards better policies faster, especially in complex environments where the original rewards might be sparse or misleading. Instead of waiting for a delayed reward, an agent can receive intermediate feedback that helps it learn the optimal behavior more efficiently.
This approach can be especially helpful when applying Q-Learning in environments with long-term delayed rewards or where sparse rewards make it hard to identify useful actions. By providing additional guidance through reward shaping, the agent has a more immediate sense of progress and can converge to the optimal policy more quickly.
Steps to Implement Reward Shaping
To effectively implement reward shaping, follow these steps:
- Define the Shaping Function: This function adds intermediate rewards to the environment. It could be based on the distance to the goal, state transitions, or other heuristics.
- Incorporate Shaped Rewards into Q-Learning: Modify the reward update rule to include the additional shaped rewards while maintaining the original objective.
- Tune the Shaping Parameters: Experiment with different shaping strategies, such as scaling the reward or using a time-decaying function, to find the most effective setup for your specific problem.
Example Reward Shaping Strategy
One simple way to implement reward shaping is by providing incremental rewards as the agent moves closer to its goal. For instance:
State | Reward |
---|---|
Start | -1 |
Close to Goal | +5 |
Goal | +10 |
Shaping rewards should be carefully designed to avoid distorting the agent's learning process or leading to unintended behavior. It’s crucial to maintain a balance between shaping and the original reward structure.
Benefits of Reward Shaping
- Faster Convergence: Helps the agent to identify the optimal policy more quickly by offering more frequent feedback.
- Improved Exploration: By providing intermediate rewards, the agent is more likely to explore a wider range of states and actions, reducing the risk of getting stuck in suboptimal solutions.
- Better Handling of Sparse Rewards: In environments with sparse or delayed rewards, shaping can guide the agent towards desirable behaviors even when direct feedback is rare.
Optimizing Q Table Updates: Key Considerations
Efficient updates of the Q-table are crucial for achieving faster and more stable learning in reinforcement learning tasks. When applying Q-learning to environments like Gym, it's essential to carefully consider the factors that influence how the Q-values are updated. These factors determine the agent's learning trajectory and the quality of the policy it derives over time.
The process of updating Q-values involves balancing exploration and exploitation. Careful adjustments to the learning rate, discount factor, and exploration strategy are key to achieving optimal performance. In this context, tuning these parameters and understanding their effects can significantly improve both convergence speed and the overall quality of the learned policy.
Key Considerations for Efficient Q Table Updates
- Learning Rate: Determines how quickly the agent incorporates new information. A high learning rate can make the agent forget previous knowledge too quickly, while a low rate may cause slow convergence.
- Discount Factor: Controls the agent’s focus on future rewards. A high discount factor encourages planning for long-term rewards, while a low one prioritizes immediate returns.
- Exploration vs. Exploitation: Balancing between exploring new actions and exploiting known good actions. Exploration can prevent the agent from getting stuck in local optima, while exploitation leverages the knowledge already gained.
Tip: Adjusting the epsilon value in epsilon-greedy strategies can fine-tune the balance between exploration and exploitation. A decreasing epsilon over time can lead to more focused exploitation as the agent gains confidence.
Strategies for Efficient Q Table Updates
- Start with a relatively high exploration rate, then gradually reduce it over time to allow the agent to focus more on exploitation as it learns.
- Implement decaying learning rates to allow the agent to learn quickly in the early stages and refine its policy as it converges.
- Use experience replay or double Q-learning to reduce the variance of the Q-value updates and stabilize learning.
Example of Q Table Update
Action | Current Q Value | Updated Q Value |
---|---|---|
Move Left | 0.3 | 0.5 |
Move Right | 0.1 | 0.25 |
Move Up | 0.4 | 0.45 |
Common Pitfalls in Q Learning and How to Avoid Them
When implementing Q Learning in reinforcement learning environments, practitioners often encounter a few common issues that can hinder the model's performance. Understanding these pitfalls and how to address them is crucial for efficient training and reliable results. Below are some of the most frequently encountered challenges and strategies to overcome them.
One of the primary issues arises from improper tuning of the learning rate, which significantly affects how quickly the agent adapts to the environment. Another common mistake is neglecting to manage the exploration-exploitation tradeoff, which can lead to suboptimal solutions if not handled carefully. Below are the most significant challenges faced by developers during the Q Learning process.
1. Incorrect Learning Rate
Choosing an inappropriate learning rate can result in the agent converging too slowly or failing to converge at all. If the rate is too high, the agent might skip over optimal solutions, while if it is too low, the learning process becomes inefficient.
Tip: Experiment with different learning rates and use decaying rates to gradually adjust the learning process as the agent becomes more confident in its actions.
2. Poor Exploration Strategy
Effective exploration is key to allowing the agent to discover the best actions. A common mistake is relying too heavily on exploitation, especially in early stages of learning, which can prevent the agent from finding optimal policies.
Tip: Implement epsilon-greedy or other exploration strategies that balance the agent's exploration of new actions with exploiting known high-reward actions.
3. Inadequate Reward Shaping
Reward functions need to be well-defined to encourage the agent to explore the desired behaviors. Poorly designed reward functions can lead to unintended behaviors or suboptimal performance.
Tip: Carefully design the reward function to align with the desired outcomes, ensuring the agent receives appropriate feedback for its actions.
4. Lack of State Representation
Improper or insufficient representation of the state space can prevent the Q-learning algorithm from learning effectively, as the agent might not have enough information to make good decisions.
Tip: Use state space abstraction or deep Q-learning to enhance state representations, allowing for better generalization across different environments.
5. Forgetting to Decay Epsilon
When using epsilon-greedy exploration, failing to decay epsilon over time can result in excessive exploration even after the agent has learned optimal policies, thus slowing down learning.
Tip: Gradually decay epsilon to balance exploration and exploitation as the agent becomes more knowledgeable about the environment.
Summary of Best Practices
Challenge | Solution |
---|---|
Incorrect Learning Rate | Adjust learning rate and use decay strategies for better convergence. |
Poor Exploration Strategy | Implement epsilon-greedy or other exploration methods to balance exploration and exploitation. |
Inadequate Reward Shaping | Design a reward function that reinforces desired behaviors and discourages undesirable ones. |
Lack of State Representation | Improve state representation using abstraction or deep learning techniques. |
Forgetting to Decay Epsilon | Ensure gradual decay of epsilon to prevent over-exploration after learning. |
Conclusion
By addressing these common pitfalls in Q Learning, developers can ensure more efficient and reliable training of reinforcement learning models. Careful tuning and thoughtful design of key components, such as the learning rate, exploration strategy, and reward function, are crucial to achieving the desired outcomes in complex environments.