How to Make Nitro Generator with Webhook
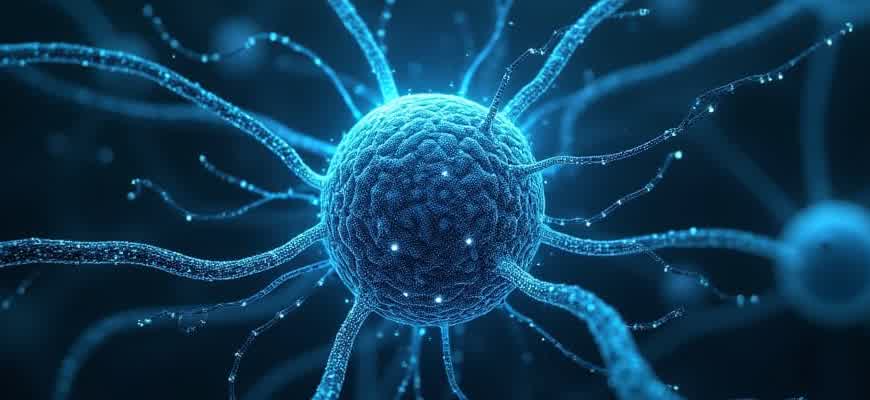
Building a custom generator for Discord Nitro codes using webhooks requires a solid understanding of API interaction and automation. The basic concept involves connecting a webhook to an automation service that can generate valid Nitro codes. The first step is to set up your webhook and create the necessary backend to handle requests.
Key Components:
- Webhook URL for receiving requests
- Backend logic to process Nitro code generation
- Automation tools like Python or Node.js
- Secure API integration for code generation
Steps to Set Up:
- Create a webhook URL from a server platform.
- Write backend logic in a programming language of choice.
- Test webhook calls and ensure correct API responses.
- Implement Nitro code validation and error handling.
Important Note: Ensure that you use valid APIs and secure your webhook to avoid misuse or abuse of your service.
Once you have the basic setup, you can refine the system by integrating validation tools and security measures to ensure the generated codes are legitimate and usable. The system will process incoming requests and return working Nitro codes based on the parameters you set up in your API call logic.
Step | Action |
---|---|
1 | Create webhook endpoint |
2 | Write backend logic for generating Nitro codes |
3 | Test the system for response handling |
Setting Up Webhooks for Nitro Generator Integration
Webhooks play a vital role in automating the process of connecting a Nitro Generator with external services. They provide a seamless communication channel between systems, triggering specific actions based on events in real-time. Proper setup is crucial for ensuring smooth data flow and reliable performance.
By configuring webhooks correctly, you can streamline tasks like sending notifications, updating databases, or triggering other automated actions in your Nitro Generator system. This guide outlines the key steps for integrating webhooks effectively.
Steps to Configure Webhooks
- Identify the event that will trigger the webhook. For example, this might be when a new user registers or when a Nitro code is generated.
- Set up the destination URL where the webhook will send the data. This URL should be hosted by the service that will process the incoming data.
- Define the data format for the webhook payload. Typically, this will be in JSON format.
- Ensure that the receiving endpoint can handle the data securely and perform the desired action based on the information received.
Webhook Integration Example
Step | Description |
---|---|
1 | Choose an event (e.g., Nitro code generation). |
2 | Provide the endpoint URL (e.g., API server where the Nitro Generator data will be sent). |
3 | Format the webhook payload (typically JSON with necessary parameters). |
4 | Test the integration by triggering the event and verifying the data is received correctly. |
Ensure that the receiving server validates the webhook data to prevent any unauthorized or malicious interactions.
Choosing the Right Platform for Webhook Support
When creating a Nitro Generator using a webhook, selecting the appropriate platform for webhook integration is essential for reliable performance and flexibility. The platform you choose should support seamless handling of HTTP requests, authentication methods, and easy integration with external services like Discord or any other third-party APIs. Different platforms offer varying degrees of complexity and features, so it is crucial to evaluate which best meets your needs based on your project's scope and requirements.
Some platforms offer built-in webhook support with minimal configuration, while others may require more customization. Understanding the strengths and limitations of each option is crucial in ensuring your webhook system functions smoothly without issues. Below are some key factors to consider when choosing a platform.
Key Features to Look for in a Platform
- Webhook Support: Ensure the platform has native support for webhook integration, allowing you to easily send and receive HTTP requests.
- Security: The platform should provide robust authentication options such as API tokens, OAuth, or other secure methods to prevent unauthorized access.
- Scalability: Consider whether the platform can handle the expected volume of webhook requests, especially if your application grows.
- Real-time Response: A low-latency response is crucial for applications requiring real-time updates or interactions.
Popular Platforms for Webhook Support
Platform | Key Features | Ease of Integration |
---|---|---|
Zapier | Easy setup, pre-built integrations with many services, automation workflows. | High |
AWS Lambda | Serverless architecture, scalable, supports custom code for complex logic. | Medium |
Discord Webhooks | Simple, direct integration with Discord, easy configuration. | High |
IFTTT | User-friendly, integrates with many third-party services, less flexibility than Zapier. | High |
Important: When selecting a platform, ensure it supports the specific API requirements of your project, such as rate limits, response time, and message formatting. This ensures smooth operation without running into unexpected restrictions.
Configuring API Endpoints for Seamless Communication
Setting up API endpoints is crucial for ensuring smooth interaction between your Nitro Generator system and external services via webhooks. The goal is to configure endpoints that can send, receive, and process data without interruption, providing a seamless flow of information. This involves establishing correct paths, managing authentication, and ensuring efficient data handling. By fine-tuning API endpoints, you can significantly enhance the performance and security of your system.
Proper API configuration starts with clearly defining endpoint routes and specifying request/response types. This will help avoid errors and improve scalability. Below are key aspects that need to be considered when configuring your API endpoints:
Key Configuration Considerations
- Route Definitions: Specify clear routes for each service your webhook will interact with.
- Authentication: Use tokens or OAuth for secure access to your endpoints.
- Data Validation: Ensure data integrity by validating incoming payloads before processing.
- Error Handling: Implement structured error responses to handle failed requests effectively.
Steps to Set Up API Endpoints
- Define Route Paths: Create specific URLs for each function, such as /generate-nitro, /verify-user, etc.
- Set Method Types: Choose appropriate methods (GET, POST, PUT, DELETE) for each route.
- Authenticate Requests: Implement token-based authentication or OAuth for added security.
- Handle Responses: Return structured responses with proper HTTP status codes (200, 400, 500).
- Implement Webhooks: Configure your endpoints to send and receive webhook notifications as needed.
Important Configuration Elements
Remember to test your API endpoints with real data and simulate errors to ensure robust handling in production environments.
Sample API Endpoint Table
Endpoint | Method | Authentication | Description |
---|---|---|---|
/generate-nitro | POST | API Key | Generates a Nitro token for the user. |
/verify-user | GET | OAuth | Verifies the user's identity before generating Nitro. |
/webhook-notify | POST | None | Sends a notification to external services. |
Handling Incoming Data: Parsing and Validation Steps
Once data is received from a webhook, it is crucial to ensure its integrity before processing. The initial step involves parsing the incoming payload, typically in JSON format, into a usable structure. This allows the application to extract the relevant details needed for further actions. Following parsing, a validation phase ensures that the data matches the expected format and values, preventing potential errors or malicious input.
The process includes several key stages, from checking for required fields to ensuring data types and ranges are appropriate. Invalid data can disrupt the functionality of the system or even lead to security vulnerabilities. Below, we outline the necessary steps to effectively parse and validate incoming data.
1. Parsing the Incoming Data
- Convert the raw payload (e.g., JSON, XML) into a structured object (such as a dictionary or map).
- Extract necessary information based on predefined keys (e.g., user ID, request type).
- Ensure the parsed structure matches expected types (e.g., strings, numbers, booleans).
2. Validation Process
- Check that all required fields are present.
- Validate data types, such as ensuring a numeric field contains a valid number.
- Apply range checks for values that have specific constraints (e.g., age must be between 18 and 100).
- Verify that no fields contain potentially harmful or unexpected characters (e.g., SQL injection prevention).
Important: Never trust incoming data without validation. Even a small data mismatch or unexpected value can cause system failures or expose vulnerabilities.
3. Example Validation Table
Field | Expected Type | Validation Rule |
---|---|---|
user_id | Integer | Must be a positive integer |
String | Must be a valid email address format | |
age | Integer | Must be between 18 and 100 |
By following these parsing and validation steps, you can ensure the reliability and security of the data received from a webhook, avoiding common pitfalls and maintaining the integrity of your application.
Testing Your Nitro Generator Workflow with Webhook Calls
Once you've set up your Nitro generator, it's essential to thoroughly test the workflow to ensure everything operates correctly. This testing phase focuses on verifying that your webhook calls are triggering the expected actions and responses from your system. Proper testing helps prevent errors that may occur when integrating with external services and ensures a smooth user experience.
In this stage, you will simulate different scenarios where the webhook is triggered, check the responses, and make sure all necessary actions, such as generating Nitro codes or sending them to the correct channels, are performed correctly. Below are the steps and techniques for testing your Nitro generator workflow effectively.
Steps to Test Webhook Calls
- Simulate webhook calls using tools like Postman or custom scripts.
- Ensure that the webhook endpoint is reachable and configured correctly in your server.
- Verify the expected payload structure and data sent with each request.
- Check the Nitro generation logic for errors or delays after receiving the webhook call.
- Review logs and responses to ensure accurate tracking and error handling.
Verifying Webhook Responses
Testing the response from the webhook is critical to ensure your Nitro generator works as intended. You should check the following:
- Success Responses: Ensure the webhook call returns a success status, typically 200 OK, when everything works as expected.
- Error Handling: Test error scenarios, such as invalid data or unreachable endpoints, to confirm that appropriate error messages are returned.
- Data Integrity: Ensure the Nitro code and other relevant data are correctly passed through the response payload.
Remember to log all responses for further debugging and verification. Consistent tracking allows you to detect potential failures early and improve your system's reliability.
Testing Table for Webhook Calls
Test Case | Expected Response | Status |
---|---|---|
Valid Webhook Call | 200 OK, Nitro code generated | Pass |
Invalid Data Format | 400 Bad Request, error message | Pass |
Webhook Timeout | 504 Gateway Timeout | Fail |
Automating Responses Based on Webhook Triggers
Webhooks provide a powerful way to automate interactions between systems. When an event occurs, a webhook sends a notification to a predefined URL, triggering an automatic response. By using this method, you can streamline processes, reduce manual intervention, and ensure consistency in how your system reacts to different inputs.
The key to effective automation with webhooks lies in understanding how to define triggers and responses that align with your workflow. By capturing specific events, such as user actions or data changes, you can set up automated processes that execute without the need for constant human oversight.
Webhook Trigger Setup
To begin automating responses based on webhook triggers, follow these steps:
- Set up your webhook endpoint on the receiving server to listen for incoming requests.
- Configure the source system to send data to this endpoint whenever specific conditions are met.
- Define the actions that should occur in response to the webhook, such as sending notifications, updating records, or triggering further processes.
Here are some key aspects to consider when automating responses:
- Event filtering: Ensure that only relevant events trigger your automation, preventing unnecessary actions.
- Data validation: Check incoming data for accuracy and completeness before triggering any responses.
- Error handling: Set up fallback mechanisms to handle any issues that arise during the automated process.
Tip: Always include logging mechanisms in your webhook processes to track events and identify potential problems during execution.
Example: Response Flow
Below is a simplified flow of an automated response system using webhooks:
Step | Action |
---|---|
1 | Webhook receives trigger from source system. |
2 | Data is validated and filtered for relevancy. |
3 | Appropriate action is triggered, such as sending an email or updating a database. |
4 | Logs are created for auditing and troubleshooting purposes. |
Implementing Error Handling and Debugging Techniques
When building a system that interacts with webhooks, especially for complex tasks such as generating Nitro codes, handling errors becomes essential. A robust error-handling strategy ensures that your application can respond gracefully to unexpected issues and provide useful feedback for troubleshooting. This includes not only detecting issues but also implementing mechanisms for recovery and preventing system crashes.
Debugging is equally critical, especially when integrating with external APIs and services. Having proper logging in place and being able to trace issues systematically can save significant time and effort. This section explores the best practices for error handling and debugging in the context of building a Nitro generator with a webhook integration.
Error Handling Strategies
- Try-Catch Blocks: Wrap critical code sections in try-catch statements to catch and handle exceptions, providing fallback behavior or logging for further analysis.
- Custom Error Messages: Always return specific error messages in response to failed operations, ensuring that users or administrators know exactly what went wrong.
- Rate Limiting: Handle cases where the webhook server might be overwhelmed by implementing retry logic with exponential backoff.
- Graceful Degradation: In case of failure, ensure the application continues to operate with reduced functionality instead of crashing entirely.
Debugging Techniques
- Logging: Set up comprehensive logging at key points of the webhook flow. Log the requests, responses, and any error states to trace issues easily.
- Unit Testing: Write unit tests to verify the behavior of individual functions that interact with the webhook. Mock external dependencies to simulate API responses.
- Real-Time Monitoring: Use monitoring tools to track the application’s performance and errors in real-time. This allows for quick detection of potential issues.
Useful Debugging Tools
Tool | Description |
---|---|
Postman | Useful for testing API endpoints and verifying webhook responses without writing additional code. |
Sentry | Real-time error tracking that helps you monitor and fix crashes in production. |
LogRocket | Tracks user interactions and API calls to help debug client-side issues. |
Note: Always ensure that your production environment has a minimal logging level to avoid performance degradation. Use more verbose logging in development or staging environments.
Monitoring Webhook Performance and Troubleshooting Issues
Once the webhook integration is set up, it is essential to continuously monitor its performance to ensure that it operates efficiently. Proper monitoring helps to identify potential issues early and take corrective actions to prevent disruptions in service. Regular checks of webhook performance will allow you to track key metrics such as response time, delivery success rate, and system load. Without this data, it can be challenging to pinpoint the cause of any failures or delays.
Additionally, troubleshooting webhook-related problems often requires a systematic approach. Identifying the root cause of an issue is critical to resolving it promptly and preventing recurring problems. This can involve checking logs, analyzing the payload, and verifying the server's status. Implementing alert systems and monitoring tools can provide valuable insights into the overall health of your webhook connections.
Key Steps for Monitoring and Troubleshooting
- Track webhook delivery rates and errors.
- Monitor response times to ensure they meet performance expectations.
- Inspect server health and resource usage regularly.
- Analyze webhook logs for detailed error reporting.
Common Troubleshooting Techniques
- Check for incorrect payload formatting or missing data.
- Ensure that the server endpoint is accessible and functioning.
- Review webhook URL configuration to prevent misrouting.
- Test the integration using mock requests to simulate real-world usage.
Important Considerations
When monitoring webhook performance, always ensure that logging is enabled. Logs can provide insights into why certain webhook requests fail, allowing you to take corrective action more quickly.
Example of Webhook Monitoring Metrics
Metric | Recommended Value |
---|---|
Response Time | Less than 500ms |
Success Rate | Over 98% |
Retry Attempts | Less than 5% |