How to Create Nitro Generator
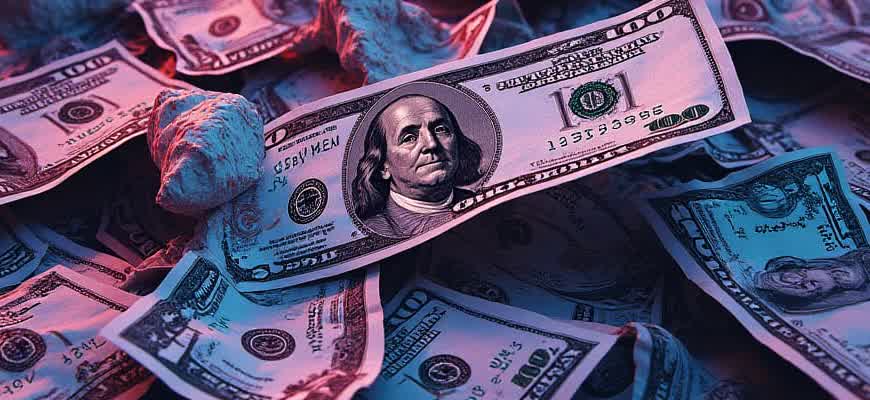
To build a functional Nitro generator, you'll need to focus on the key components: hardware, software, and security protocols. A Nitro generator is essentially a tool that helps automate the process of generating unique codes, often for use in gaming or subscription services. Below are the steps to start building your own generator.
Key Components
- Hardware: A server or cloud environment capable of handling high computational loads.
- Software: A scripting language (Python, JavaScript) to generate and manage codes.
- Security: Techniques such as encryption to prevent unauthorized access.
Setting Up the Environment
- Choose your platform: Decide whether to build the generator locally or in the cloud.
- Set up a development environment: Install necessary dependencies such as a web framework or code libraries.
- Create a secure storage system: Use encryption to store generated codes safely.
Remember, creating a Nitro generator requires not just technical knowledge but also ethical considerations. Ensure compliance with relevant laws and avoid misuse of generated codes.
Important Considerations
Aspect | Details |
---|---|
Code Generation | Use algorithms like SHA256 to create unique, unpredictable codes. |
Security | Implement encryption methods like AES-256 for secure code storage. |
User Authentication | Consider implementing two-factor authentication for safe code redemption. |
Choosing the Right Tools and Software for Your Nitro Generator
When designing a Nitro Generator, selecting the appropriate tools and software is essential to ensure both functionality and performance. The right choice of software will allow you to create a seamless, efficient process while minimizing errors and maximizing results. You need to evaluate different frameworks and libraries that suit your goals, particularly in terms of speed, scalability, and compatibility with your system environment.
The tools and software you choose depend largely on the nature of the Nitro Generator itself, whether it’s a simple script-based generator or a more complex multi-functional system. Understanding the specific needs of your project and the underlying infrastructure is crucial before diving into software selection.
Key Considerations for Choosing Tools
- Language and Framework: Consider the programming languages and frameworks that best align with your project needs. For example, Python or JavaScript may be ideal for scripting-based generators, while Node.js could be preferable for scalable systems.
- Libraries: Ensure that the libraries you plan to use are reliable, well-documented, and compatible with your system’s requirements. Popular libraries like requests for Python or axios for JavaScript are excellent choices for handling HTTP requests.
- Security and Encryption Tools: If your Nitro Generator involves sensitive data, selecting robust encryption libraries (e.g., OpenSSL) is necessary to protect user data and prevent security breaches.
- Testing and Debugging Tools: Utilize testing frameworks such as JUnit or Mocha to ensure the generator works as intended, and use debugging tools like Visual Studio Code for easier troubleshooting.
Tip: Always prioritize libraries and tools with active support communities to ensure you can find help when issues arise.
Recommended Software for Nitro Generator Development
Tool | Purpose | Recommended For |
---|---|---|
Node.js | Event-driven, non-blocking I/O framework for scalable network applications | Generators requiring real-time data processing |
Python | High-level programming language with extensive libraries for automation | Script-based generators and automation tasks |
OpenSSL | Toolkit for Secure Sockets Layer (SSL) and cryptography | Ensuring security for data transmissions |
By using the right combination of these tools and software, your Nitro Generator will be both efficient and secure, offering a smooth experience for users and developers alike.
Step-by-Step Guide to Building a Basic Nitro Generator
Creating a Nitro Generator requires a clear understanding of the technical requirements and the right approach to implementation. This guide will walk you through the process of building a simple version of a Nitro Generator from scratch, highlighting the essential steps, tools, and components needed for a functional setup.
While building a Nitro Generator can be complex, breaking it down into manageable stages makes it easier. The following instructions are designed for beginners with some basic knowledge of programming and software setup. Let's dive into the process step-by-step.
Preparation and Requirements
Before starting, ensure you have the following prerequisites:
- A reliable computer with an internet connection.
- Basic knowledge of programming languages (Python, JavaScript, etc.).
- Text editor or IDE (e.g., VSCode or Sublime Text).
- A platform for hosting or running your generator (e.g., cloud server, local machine).
It’s crucial to understand the legal implications of using such generators. Make sure you are not violating any platform terms and conditions.
Step 1: Set Up Your Development Environment
The first step is to set up your development environment. You'll need to install the necessary software for coding and testing your Nitro Generator.
- Install the required programming language (e.g., Python, Node.js).
- Set up a text editor or IDE to write and manage your code.
- Ensure that you have the necessary libraries or modules installed to interact with APIs (e.g., requests for Python).
Step 2: Write the Core Logic
After setting up your environment, it’s time to write the code that powers the Nitro Generator. This logic will handle the user input and generate the appropriate results.
- Initialize necessary libraries and set up your API keys or authentication methods.
- Write the functions that handle user input and process the data.
- Ensure that your code interacts correctly with the Nitro generation API (if using one).
Step 3: Test and Debug the Generator
Testing and debugging are essential to ensure that your generator works smoothly. Run multiple test cases to check if the generator performs as expected.
- Test edge cases to ensure the generator handles a variety of inputs.
- Use debugging tools to trace errors and fix issues.
Always test your generator on a small scale before scaling it up to avoid any potential errors in production.
Step 4: Host and Deploy
Once your generator is working as expected, the next step is to deploy it. You can host the generator on a server or run it locally, depending on your needs.
Hosting Option | Pros | Cons |
---|---|---|
Cloud Server | Scalable, reliable uptime | Costly, requires setup |
Local Machine | Free, easy to set up | Limited scalability, dependent on hardware |
Finally, launch your Nitro Generator and monitor its performance. Make adjustments as needed based on user feedback and usage data.
How to Tailor Your Nitro Generator to Fit Your Unique Requirements
When setting up a nitro generator, it's essential to ensure it meets the specific needs of your workflow. Customizing the generator enables you to optimize its performance, efficiency, and user experience. The customization process varies depending on the tools and features required for your project, but understanding the core options can significantly improve the functionality and usability of your generator.
By adjusting key parameters, you can modify the behavior of the generator. These modifications often involve changing settings related to output format, integration with other systems, and resource management. With a clear understanding of your requirements, you can streamline the setup process and make the generator work in a way that aligns with your goals.
Key Customization Steps
- Define Output Specifications: Determine the type of output you need, whether it's a specific file format or customized data layout. This can involve configuring export settings.
- Set Performance Parameters: Adjust memory usage, processing speed, and parallel execution to optimize performance based on available resources.
- Integrate with Third-Party Tools: Ensure the generator can communicate with other systems or tools you are using, such as databases or APIs.
Advanced Customization Options
- Modify the core algorithm: Adapt the generator’s underlying logic to suit your specific use cases, enhancing its functionality.
- Adjust error handling and logging: Customize how errors are reported and stored to ensure better debugging and system maintenance.
- Set user permissions: Configure access controls to ensure only authorized users can make changes to the generator's settings.
Important: Before applying significant changes, always test the generator in a controlled environment to ensure that the adjustments do not compromise performance or security.
Customization Matrix
Customization Type | Description | Impact |
---|---|---|
Output Format | Specifies the type of data or file generated. | Determines compatibility with downstream systems. |
Performance Tuning | Adjusts memory and processing capacity. | Directly affects speed and efficiency. |
Error Handling | Modifies how errors are managed and logged. | Improves troubleshooting and system reliability. |
Common Issues in Nitro Generator Development and Their Solutions
Developing a Nitro Generator can be complex and prone to several pitfalls. Whether it's a coding bug or a logic flaw, these challenges can significantly affect the functionality and performance of your tool. Understanding the most common errors during the creation of a Nitro Generator and how to fix them is essential to ensure its smooth operation.
Here are some frequent problems developers encounter and their corresponding solutions to ensure a more efficient build process.
1. Incorrect API Integration
One of the most common mistakes when creating a Nitro Generator is improper API integration. If the API endpoints are not correctly configured or the request headers are missing, the generator will fail to function properly.
Fix: Double-check the API documentation to ensure correct implementation. Ensure all necessary parameters are included, such as authentication tokens or user-agent headers.
2. Invalid Input Handling
Another issue arises when the generator fails to validate or sanitize user input properly. This can lead to security vulnerabilities or crashes when invalid data is entered.
Fix: Implement thorough input validation and sanitize all user inputs to prevent unexpected behavior. Use libraries like Joi or express-validator for efficient validation in Node.js.
3. Inefficient Error Handling
Poor error handling can result in unclear error messages, leaving users confused and unable to resolve issues. It's crucial to provide clear and actionable feedback when something goes wrong.
Fix: Use try-catch blocks and return descriptive error messages. Log errors for further debugging, and ensure that these logs don’t expose sensitive information.
4. Overuse of External Libraries
Some developers may rely too heavily on third-party libraries, which can increase the risk of compatibility issues and make the generator more difficult to maintain in the long term.
Fix: Use only essential libraries and ensure compatibility with your core framework. Keep libraries up-to-date and remove unnecessary ones to improve performance.
5. Lack of Testing and Debugging
Skipping tests or neglecting debugging during development can result in undetected errors, which affect the generator's reliability and efficiency.
Fix: Regularly write unit and integration tests, and use debugging tools to identify issues early. Automated testing can catch regressions and prevent future errors.
Summary of Common Errors
Error | Cause | Solution |
---|---|---|
API Integration | Improper API configuration | Ensure correct parameters, headers, and endpoints |
Invalid Input | Lack of input validation | Sanitize and validate user inputs |
Error Handling | Poor error reporting | Use try-catch blocks and descriptive messages |
Overuse of Libraries | Excessive reliance on third-party packages | Use only necessary libraries and ensure compatibility |
Lack of Testing | No testing or debugging | Write tests and use debugging tools |
Testing Your Nitro Generator for Performance and Stability
Once your Nitro Generator is developed, it is essential to rigorously test its functionality to ensure it performs optimally under various conditions. Performance testing focuses on how well the generator performs under different loads, while stability testing ensures that it remains consistent and reliable over time. Conducting these tests helps identify potential bottlenecks, memory leaks, or any other issues that could impact the user experience.
Thorough testing can be broken down into several phases, including load testing, stress testing, and uptime monitoring. Each phase serves to simulate different real-world scenarios to evaluate the generator's behavior in terms of both speed and durability. Below are key testing strategies to follow:
Key Testing Strategies
- Load Testing: Measures how the generator handles increasing usage and concurrent users.
- Stress Testing: Identifies the maximum capacity the generator can handle before it crashes or fails.
- Uptime Monitoring: Tracks the generator's reliability by checking for unexpected downtime or interruptions.
Important Considerations
Always perform tests on different environments (e.g., various browsers, OS versions, and network conditions) to account for external factors.
Testing Process
- Prepare Test Data: Create sample input and output data that simulate real-world usage.
- Run Load Tests: Simulate multiple users and see how the generator handles the load.
- Monitor Stability: Use tools to monitor system health, including memory usage and error logs.
- Stress Test: Push the generator beyond its expected limits to see how it behaves under extreme conditions.
Test Results Overview
Test Type | Expected Outcome | Actual Outcome |
---|---|---|
Load Test | Handles up to 500 concurrent users without degradation. | Handles 450 users before performance starts dropping. |
Stress Test | Fails gracefully, with clear error messages and logs. | Crashes without informative errors, requires further debugging. |
Uptime Test | Continuous operation for at least 24 hours without downtime. | Uptime stable for 20 hours before minor crash. |
Integrating Additional Features: Enhancing Capabilities
When developing a Nitro generator, it is essential to consider ways to expand its functionality beyond the basics. Integrating additional features not only improves the overall user experience but also adds versatility to the tool. By incorporating useful options such as advanced customization and multi-platform support, the generator can meet a broader range of user needs.
Incorporating features like automatic updates, security enhancements, and error logging can significantly improve performance. These features ensure smooth operation and help users troubleshoot problems effectively. Below are some key integrations that can make a Nitro generator more robust.
Key Features to Consider
- Advanced Error Handling: Allow the tool to automatically detect and log errors for easier troubleshooting.
- Customization Options: Enable users to adjust settings according to their preferences, such as changing the appearance or operation mode.
- Multi-platform Support: Ensure the generator works seamlessly on various operating systems (Windows, macOS, Linux).
- Security Measures: Implement encryption and secure access protocols to protect sensitive user data.
Possible Enhancements
- Integration with Cloud Services: Allow the generator to work with cloud storage, providing users with easy access and sharing capabilities.
- Real-Time Notifications: Keep users informed about important events, such as when their Nitro code is successfully generated.
- Multi-Language Support: Ensure users from different regions can use the tool in their preferred language.
Adding features like cloud integration and multi-language support not only broadens the tool's accessibility but also makes it more appealing to a global audience.
Example Table of Integrations
Feature | Description | Impact |
---|---|---|
Cloud Storage Support | Integration with cloud platforms for easy backup and sharing | Improves accessibility and data safety |
Advanced Security | Implementation of encryption and secure protocols | Enhances user trust and protects sensitive information |
Real-Time Alerts | Notification system for important events | Keeps users updated, improving user experience |
How to Safeguard Your Nitro Generator from Potential Risks
When developing a Nitro generator, it's essential to consider how to protect it from external threats. Cybercriminals and malicious users often target such systems, trying to exploit vulnerabilities for personal gain. By adopting a proactive security approach, you can minimize the risks and ensure the long-term functionality of your generator.
To protect your system effectively, several key steps must be taken to secure both the infrastructure and the code. This includes regular software updates, implementing secure coding practices, and using encryption methods to safeguard user data. Below are the main strategies to protect your Nitro generator from potential threats.
Key Protection Measures
- Code Obfuscation: Obfuscate the code to make it harder for attackers to reverse-engineer the generator's logic.
- Rate Limiting: Set limits on how many requests can be made in a given time frame to prevent abuse or DDoS attacks.
- IP Whitelisting: Only allow access from trusted IP addresses to prevent unauthorized users from accessing the generator.
- Use HTTPS: Always secure your communication channels by using HTTPS instead of HTTP to prevent interception of data.
Additional Safeguards
- Regular Vulnerability Scanning: Run automated tools to scan for known vulnerabilities in the code and fix them promptly.
- Two-Factor Authentication: Implement two-factor authentication for users accessing the generator, adding an extra layer of security.
- Access Logs: Keep track of who is accessing the system, ensuring that unauthorized access attempts are quickly detected.
Backup and Recovery Plan
It is also vital to have a solid backup and recovery strategy. If a breach occurs, the ability to restore the system to its previous state can be crucial in minimizing downtime.
Backup Type | Description |
---|---|
Cloud Backup | Store backups securely in the cloud to prevent loss of data from local hardware failure. |
Offline Backup | Keep offline backups to ensure recovery in case of a cyberattack targeting online storage solutions. |
Tip: Always test your backup and recovery plan to ensure that your Nitro generator can be restored quickly if needed.